The Complete Computer Science Master Class Bundle
What's Included
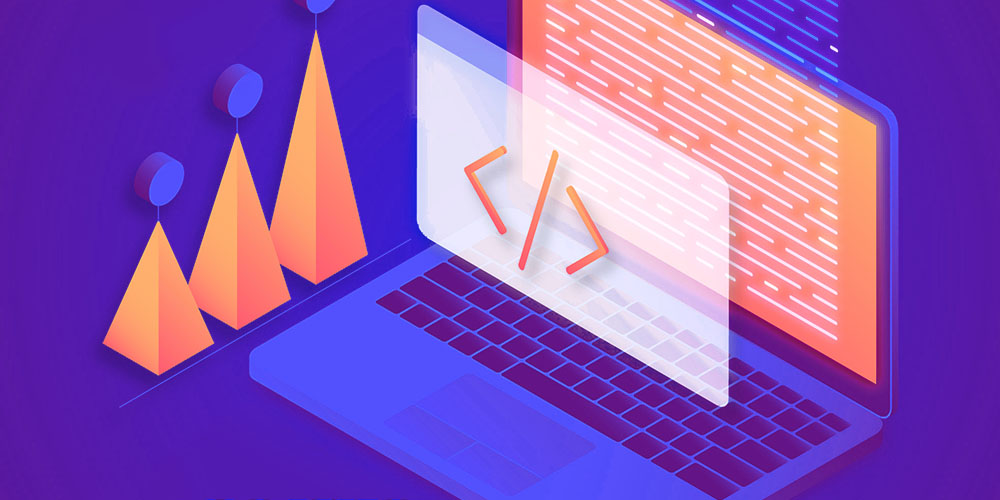
The Complete C# Masterclass
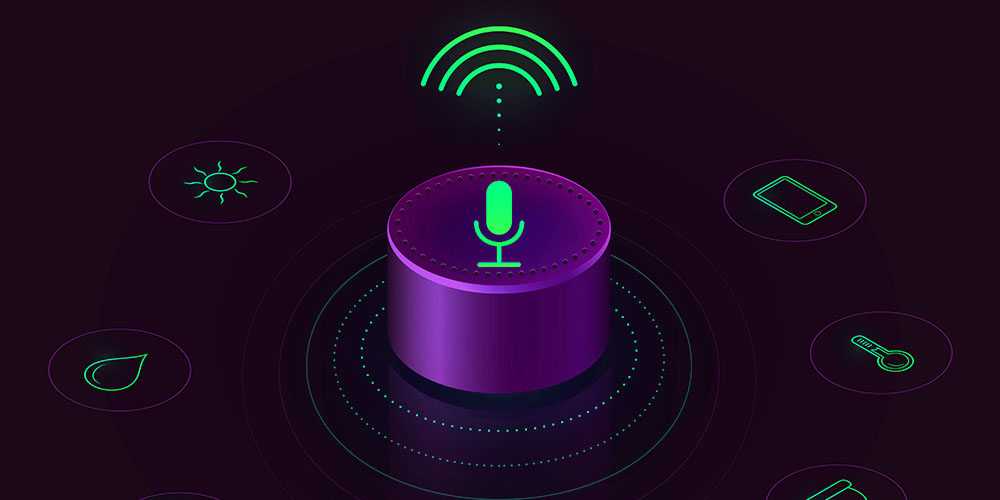
Building Voice Apps Using Amazon Alexa
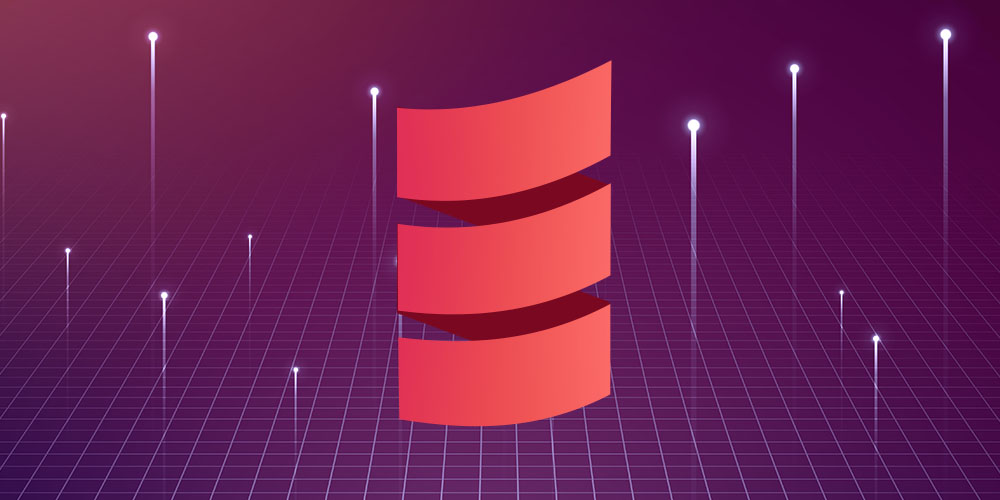
Learn By Example: Scala
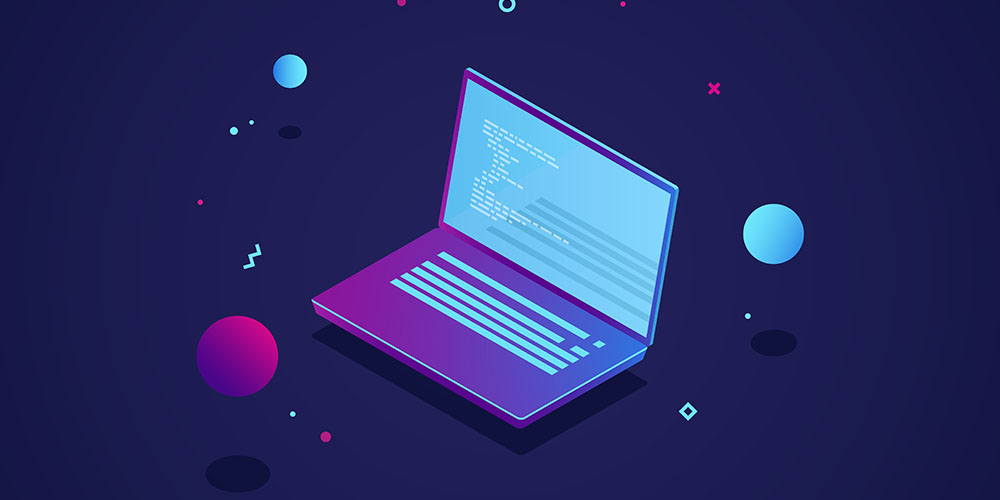
Software Testing Omnibus
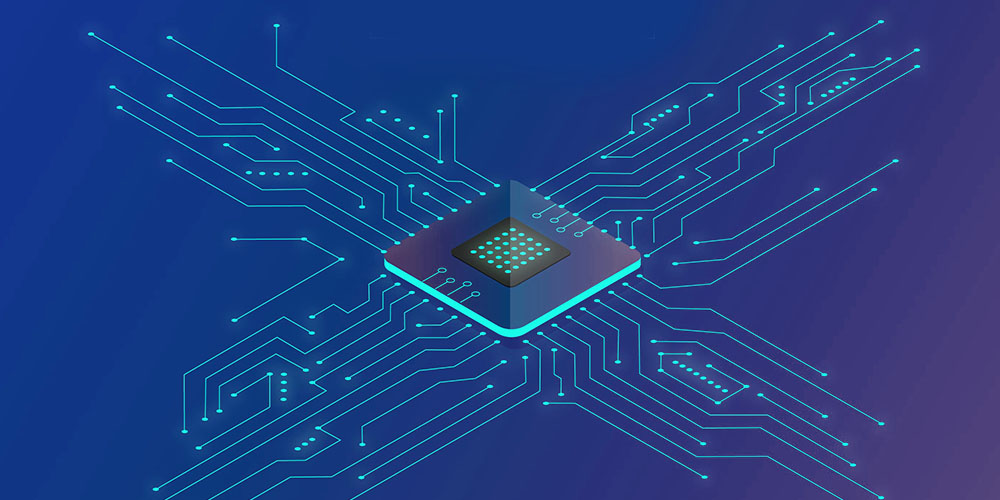
Arduino IoT Cloud Bootcamp
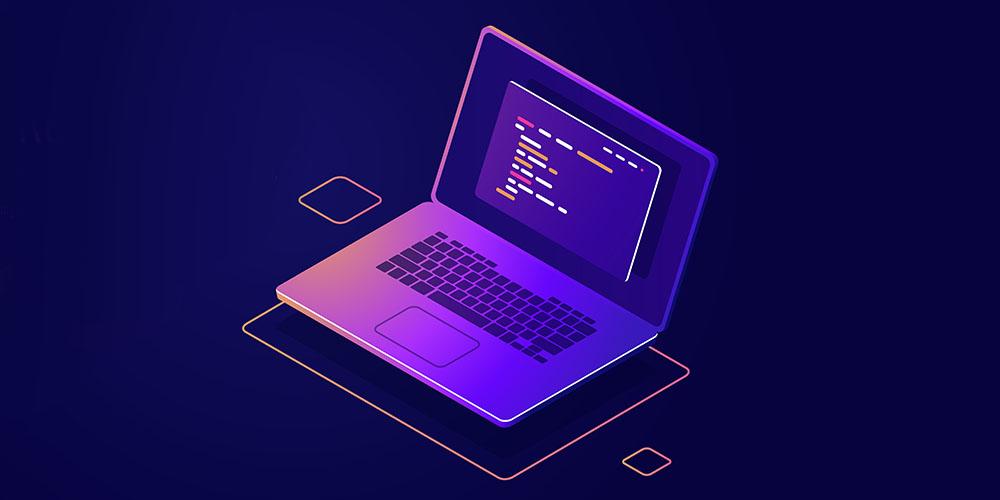
The 2019 JavaScript Developer Bootcamp
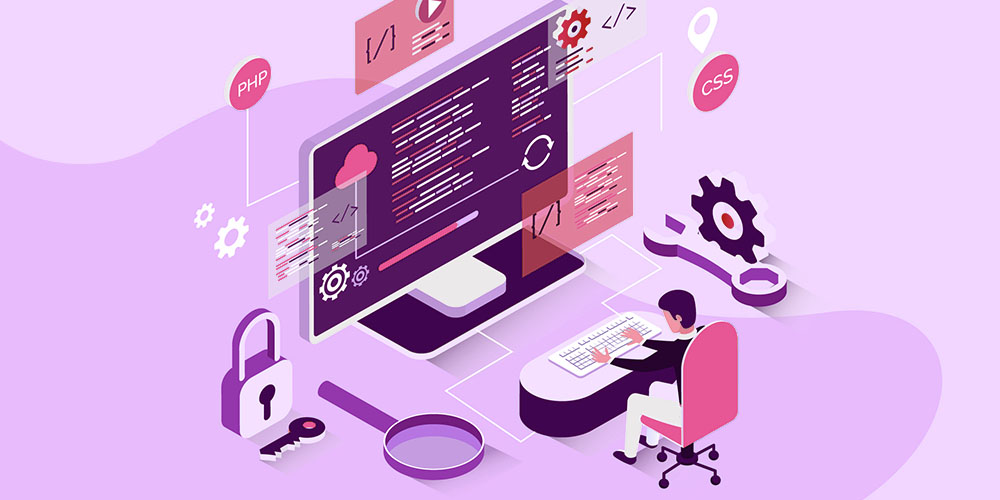
Start Golang Programming Today & Become a Master of Google Go
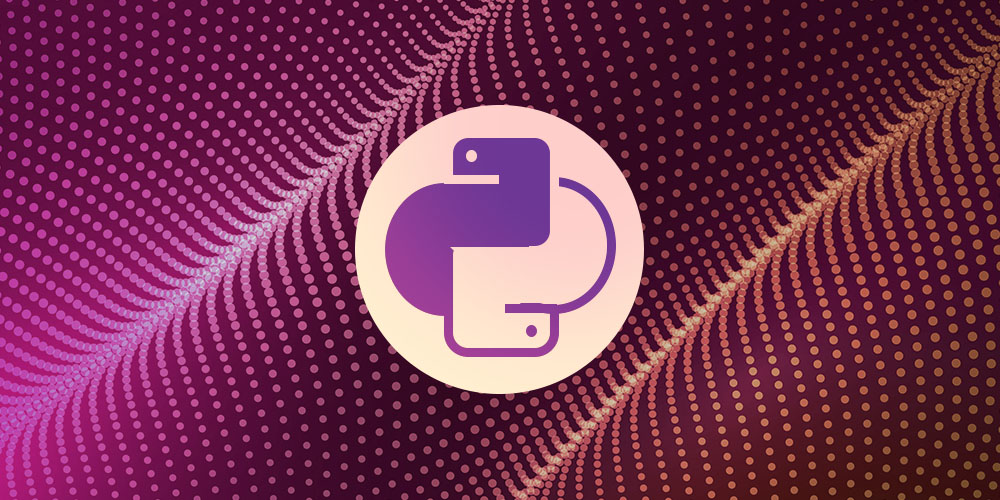
Start Python 3 Programming Today
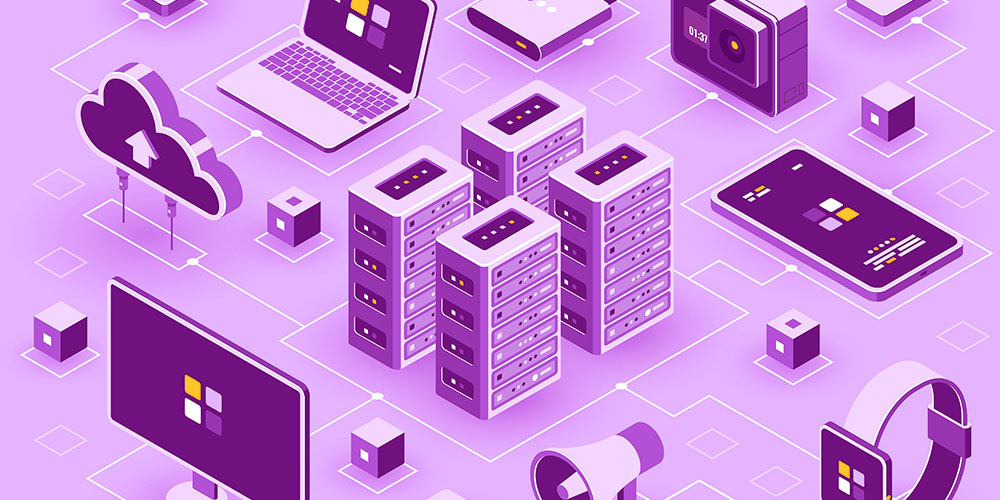
The Complete PHP MySQL Professional Course with 5 Projects
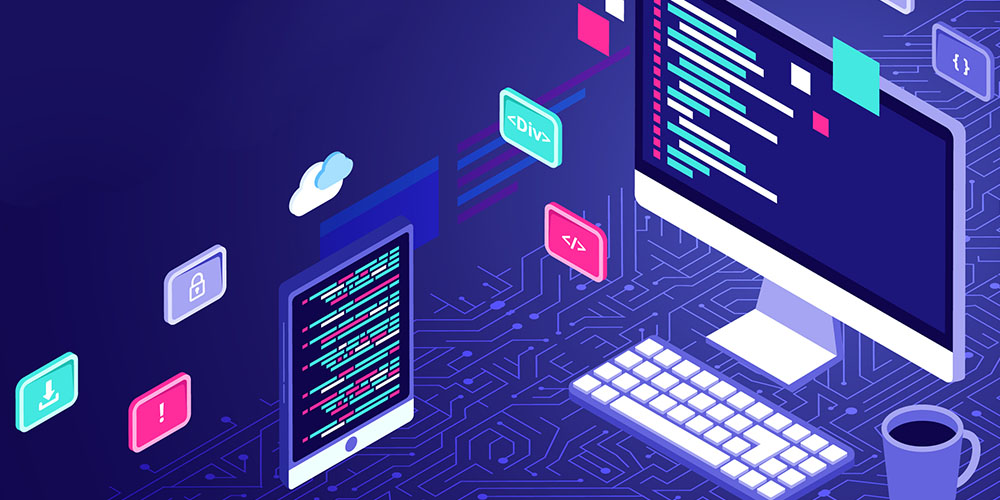
Break Away: Programming & Coding Interviews
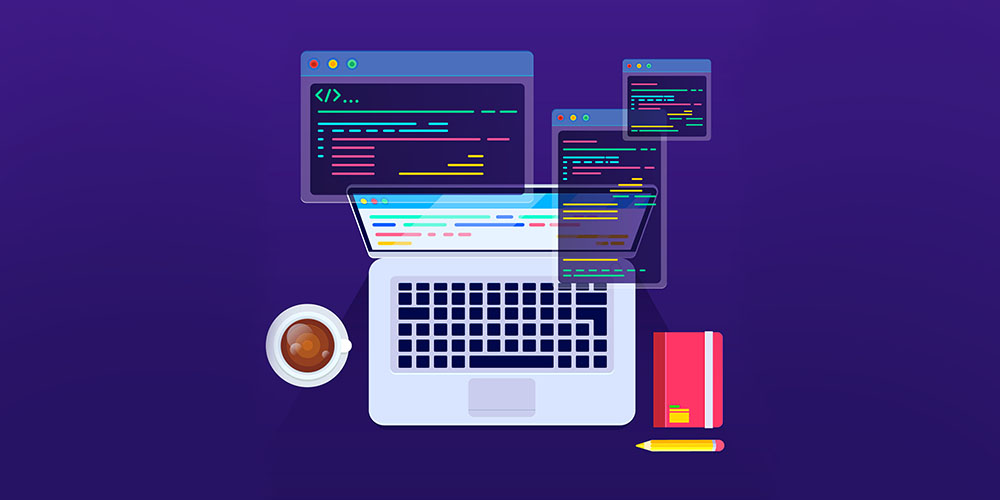
From 0 to 1: Data Structures & Algorithms in Java
Terms
- Unredeemed licenses can be returned for store credit within 30 days of purchase. Once your license is redeemed, all sales are final.
Ikem Nwokike
It's everything I needed to get started, it's well structured to put one through the paces and equip one with competent skills
luke lorusso
Found this deal after purchasing something else from the store. Definitely getting a good value. Worthwhile purchase.
Joseph Ruel
Had started a different coding class and realised it was missing many elements to cover the topic for real so i tried huff shiet and this is perfect. Point by point without omiting anything,, they guide you through code. Excellent course.
Ela Lubecka
Most of the courses were created in 2017, which should be mentioned before purchasing. Pretty useful for the beginners, but for someone who's looking after revision/update of their skills, I would recommend different bunch.
Winston Hibler
Awesome deal
Kayla Preece
Coronavirus has me at home learning new things. I haven't looked at this product yet but I am being forced to rate this product so take it for what its worth.